If you're using a PCRE flavor, the following one could suit your needs (formatted for readability):
^(?:
((?=.*d))((?=.*[a-z]))((?=.*[A-Z]))((?=.*[^a-zA-Z0-9]))|
(?1) (?2) (?3) |
(?1) (?2) (?4) |
(?1) (?3) (?4) |
(?2) (?3) (?4)
).{4,8}$
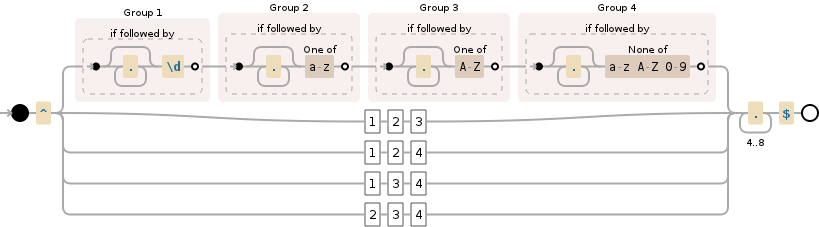
One-lined:
^(?:((?=.*d))((?=.*[a-z]))((?=.*[A-Z]))((?=.*[^a-zA-Z0-9]))|(?1)(?2)(?3)|(?1)(?2)(?4)|(?1)(?3)(?4)|(?2)(?3)(?4)).{4,8}$
Demo on Debuggex
JavaScript regex flavor does not support recursion (it does not support many things actually). Better use 4 different regexes instead, for example:
var validate = function(input) {
var regexes = [
"[A-Z]",
"[a-z]",
"[0-9]",
"[^a-zA-Z0-9]"
];
var count = 0;
for (var i = 0, n = regexes.length; i < n; i++) {
if (input.match(regexes[i])) {
count++;
}
}
return count >=3 && input.match("^.{4,8}$");
};
与恶龙缠斗过久,自身亦成为恶龙;凝视深渊过久,深渊将回以凝视…